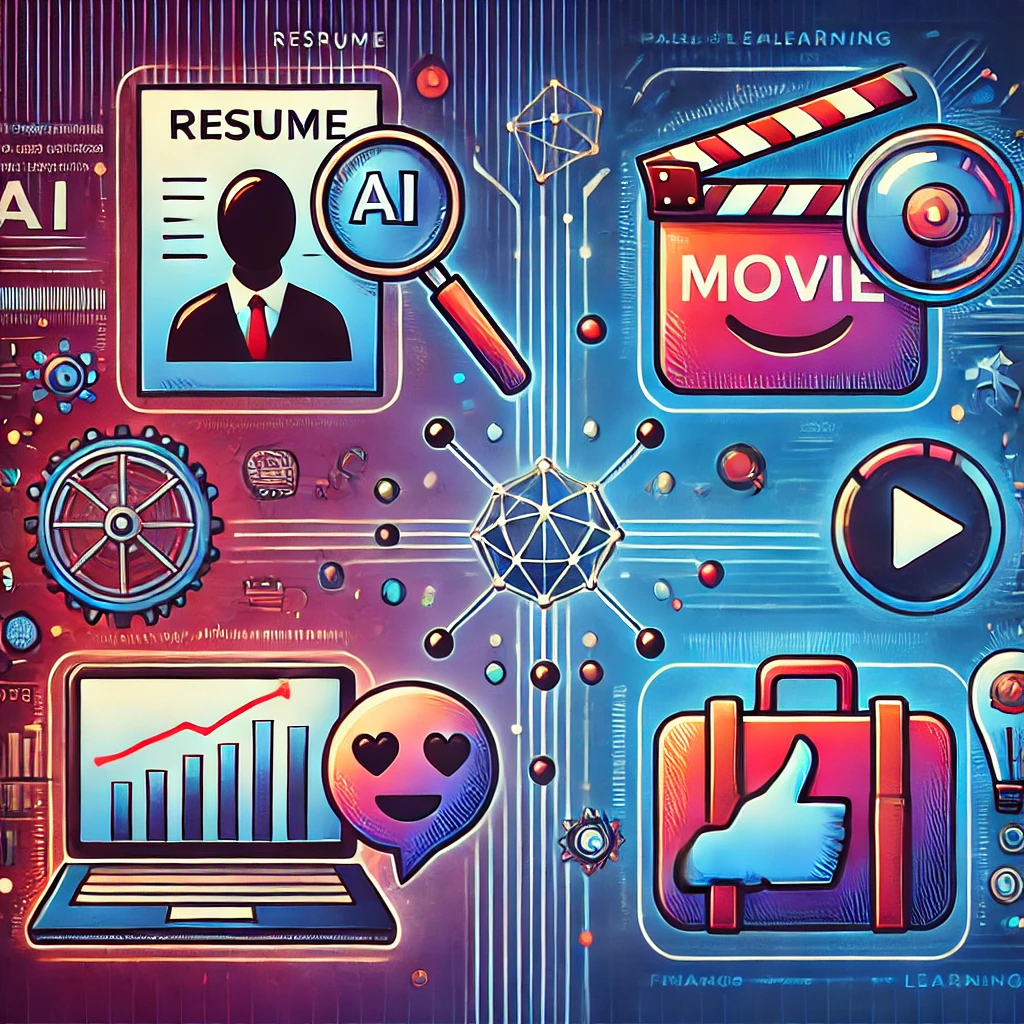
If you’re looking to improve your skills in artificial intelligence (AI) or add some new projects to your portfolio without spending weeks on them, you’re in the right place. In this article, we’re going to take a look at 5 projects you can build fast using Python.
From time to time I have been approached by aspiring developers who are either early in their career or are working toward landing their first role in data science or machine learning (ML). These developers ask, in one form or another, something like “I feel like I need a portfolio but I just don’t know where to start.” I always have the same answer: make something up. It’s really that simple.
Think of something that interests you, and frame a project around an insight or a feature that you would have fun implementing. Enjoy sports? Train a ML model to predict the outcome of games. Enjoy hiking? Build a trail recommendation system. Enjoy history? Create a historical text analysis Natural Language Processing (NLP) model.
Now let’s explore 5 other projects you can build using Python. Each project includes a brief description and example Python code to get you started.
Project 1: AI Resume Analyzer
The AI Resume Analyzer helps job applicants by comparing resumes to job descriptions and provides a match score. This project uses natural language processing (NLP) to extract relevant keywords from both the resume and the job posting, which is useful for both job seekers and hiring professionals.
Key Concepts:
- Text processing with NLP
- Document similarity
- Cosine similarity
Python Code Snippet:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
def resume_match(resume_text, job_description):
vectorizer = TfidfVectorizer()
vectors = vectorizer.fit_transform([resume_text, job_description])
similarity = cosine_similarity(vectors[0:1], vectors[1:2])
return similarity[0][0]
# Example usage
resume = "Experienced data scientist with skills in Python, machine learning, SQL..."
job = "Looking for a Python developer with machine learning experience..."
print(f"Match Score: {resume_match(resume, job)}")
Project 2: Movie Genre Predictor Using Plot Summaries
In this project, you’ll build a movie genre prediction tool that takes in a movie plot summary and predicts its genre. Now you might be thinking, what is the use of doing this considering every movie you can think of already has an assigned genre. Remember – the primary goal of doing projects to boost your portfolio and skillset lies in the journey and not the destination. Showcasing your skills and gaining exposure to varying types of models and tools is the idea here. This project helps you dive into text classification, one of the fundamental tasks in NLP, using Python.
Key Concepts:
- Text classification
- Supervised learning
- Feature extraction
Python Code Snippet:
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.naive_bayes import MultinomialNB
# Example data: load your dataset of plot summaries and genres
plot_summaries = ["A hero saves the world", "A love story in Paris...", "..."]
genres = ["Action", "Romance", "..."]
X_train, X_test, y_train, y_test = train_test_split(plot_summaries, genres, test_size=0.2)
vectorizer = CountVectorizer()
X_train_vec = vectorizer.fit_transform(X_train)
model = MultinomialNB()
model.fit(X_train_vec, y_train)
Project 3: Smart Expense Categorizer
The Smart Expense Categorizer helps automatically classify expenses based on transaction descriptions, making it a perfect tool for personal finance applications. This project leverages machine learning and NLP to recognize and categorize different types of expenses.
Key Concepts:
- Data preprocessing
- Classification with logistic regression
- NLP for transaction categorization
Python Code Snippet:
import pandas as pd
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.linear_model import LogisticRegression
# Example transaction data
data = pd.DataFrame({
'Transaction': ['Starbucks Coffee', 'Amazon Purchase', 'Uber Ride'],
'Category': ['Food & Drink', 'Shopping', 'Transport']
})
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(data['Transaction'])
model = LogisticRegression()
model.fit(X, data['Category'])
# Example prediction
new_transaction = ["Uber Eats"]
print(model.predict(vectorizer.transform(new_transaction)))
Project 4: Real-Time Emotion Detection from Speech
Changing directions here and working in a project that deals with audio. Emotion detection from speech is an interesting AI project that involves analyzing audio data to classify emotions with labels like happy, sad, or angry. You’ll gain experience working with audio signals and building classification models.
Key Concepts:
- Audio signal processing
- Emotion classification
- Neural networks for speech analysis
Python Code Snippet:
import librosa
from sklearn.model_selection import train_test_split
from tensorflow.keras import Sequential
from tensorflow.keras.layers import Dense
# Load audio data
def extract_features(audio_file):
y, sr = librosa.load(audio_file)
return librosa.feature.mfcc(y=y, sr=sr)
X_train, X_test, y_train, y_test = train_test_split(audio_features, emotions)
# Build a simple neural network
model = Sequential([
Dense(64, activation='relu', input_shape=(X_train.shape[1],)),
Dense(32, activation='relu'),
Dense(3, activation='softmax')
])
Project 5: AI Travel Itinerary Planner
The AI Travel Itinerary Planner uses a simple recommendation system to generate travel plans based on user preferences like destination, budget, and interests. This project introduces the basics of optimization and recommendation systems.
Key Concepts:
- Recommendation systems
- Data personalization
- Optimization for preferences
Python Code Snippet:
import random
def generate_itinerary(preferences):
destinations = ["Paris", "Tokyo", "New York", "Sydney"]
selected_destination = random.choice(destinations)
return f"Destination: {selected_destination}, Activities: {preferences['interests']}"
# Example usage
user_preferences = {'budget': 1000, 'interests': ['museums', 'restaurants']}
print(generate_itinerary(user_preferences))
Conclusion
I have been on both sides of the interview table for various data and machine learning roles at a Fortune 500 company and in my experience having a portfolio isn’t always necessary as part of the hiring process; however, I would still recommend having a handful of projects in a portfolio and keeping it up to date for two reasons:
- If a hiring manager requests a portfolio you have one ready to go.
- Creating the portfolio provides experience you likely didn’t have before which improves your skillset – remember, it’s about the journey!
Each of the projects above offer a hands-on way to sharpen your AI and Python skills while building something practical and impressive for your resume or CV. The ability to showcase the skills required to build these AI application is a valuable asset in today’s job market. Plus, they’re great conversation starters for interviews and can demonstrate your ability to solve real-world problems through code.
Working on projects like these enhances you understanding of AI and helps you develop essential skills for career growth, while providing quick boosts to your resume.
Check out Kaggle for additional datasets, notebooks, and tutorials focused on machine learning and AI projects. You can also join competitions to apply your skills to real-world problems, get feedback from the community, and learn from top data scientists.
If you’re new to Python and are looking for a quick start, take a look at our Learning Python Series.