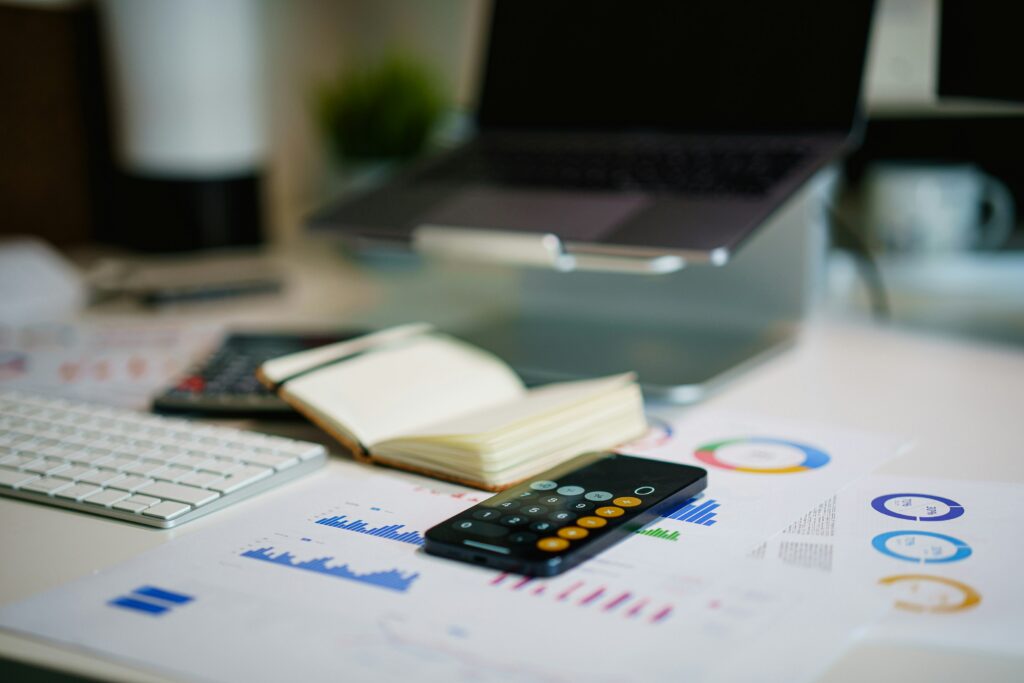
In this eighth installment of our Learning Python series, we’ll dive into NumPy basics, a powerful library for numerical computing in Python. NumPy is essential for data manipulation and analysis, especially when dealing with large datasets.
The Jupyter notebook file associated with this blog post, which includes all of this information, can be found here. We encourage you to code along in your own Jupyter notebook file and use the linked notebook as a guideline.
Creating and Manipulating Arrays
NumPy arrays are more efficient than Python lists for numerical operations. To start using NumPy, you first need to install it. If you’ve been following along then this step will already be done as outlined in our Learning Python: Part 7 – Introduction to Libraries NumPy and Pandas post.
# Install NumPy (if not installed)
!pip install numpy
# Import NumPy
import numpy as np
# Create a NumPy array
array = np.array([1, 2, 3, 4, 5])
print("Array:", array)
Basic Array Operations
NumPy allows for a variety of operations on arrays, including mathematical computations and statistical operations.
# Array operations
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Addition
sum_array = array1 + array2
print("Sum:", sum_array)
# Mean
mean_value = np.mean(array1)
print("Mean:", mean_value)
# Reshape
reshaped_array = array1.reshape((3, 1))
print("Reshaped Array:\n", reshaped_array)
Practical Examples with NumPy
Let’s consider a practical example where NumPy can be useful. Suppose you need to analyze a dataset of temperatures:
temps = np.array([22.5, 24.0, 22.7, 24.3, 27.3])
average_temp = np.mean(temps)
print("Average Temperature: ", average_temp)
Conclusion
By utilizing NumPy’s operations, you can quickly perform data analysis and manipulation tasks.
There are a ton of additional resources available for diving deeper into all that NumPy has to offer. Here are some of the important ones:
Official NumPy Documentation: The most comprehensive resource for learning about all the features of NumPy. It’s great for reference and in-depth reading.
NumPy Documentation
NumPy Tutorial by Real Python: An easy-to-follow tutorial that covers the basics of NumPy and some more advanced topics in a beginner-friendly way.
Real Python NumPy Tutorial
NumPy for Data Science Beginners: A complete beginner guide to NumPy, ideal for those just starting out in data science.
NumPy Guide by Analytics Vidhya