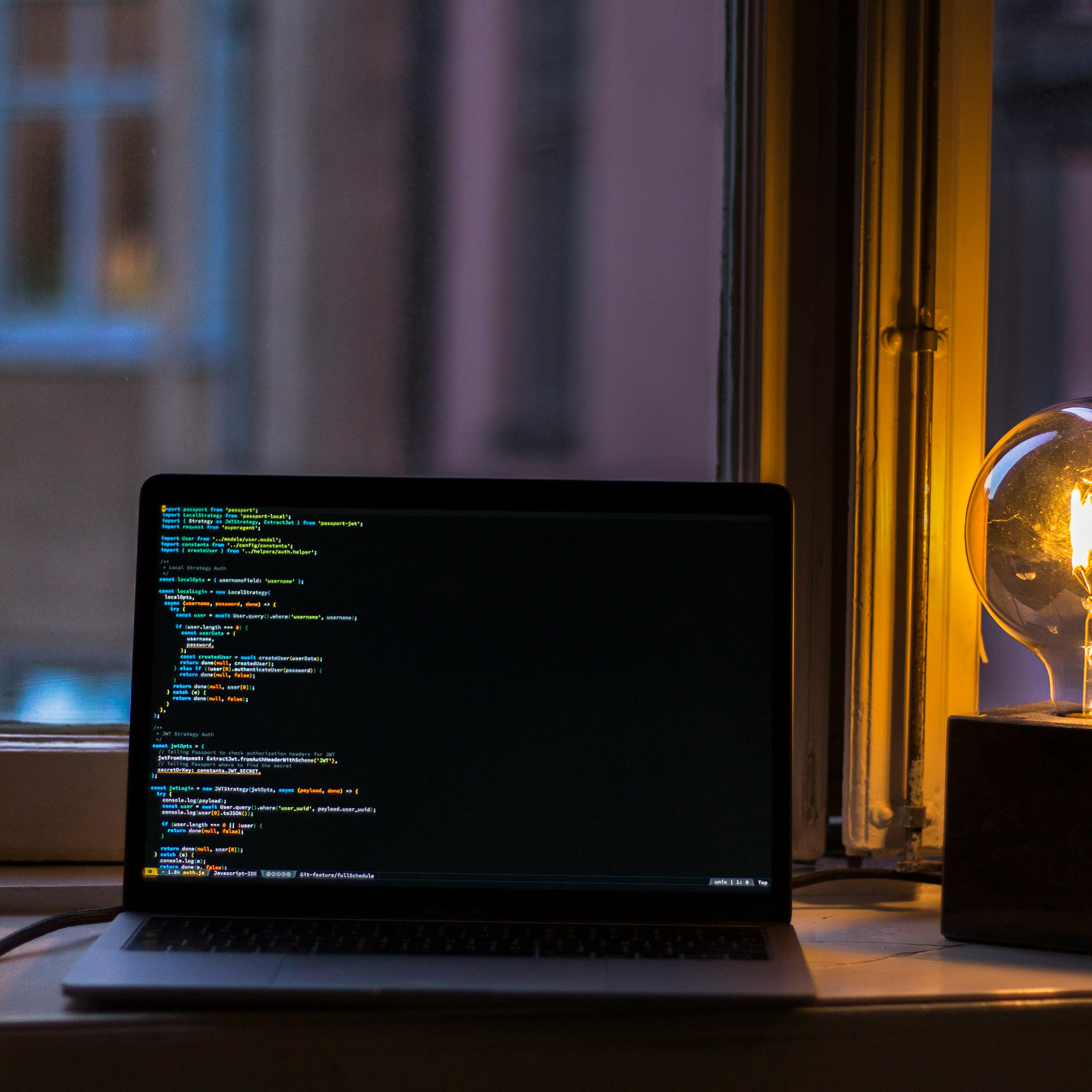
Welcome to the fifth part of our Learning Python series. In this post, we’ll dive into some foundational programming concepts that are essential for any aspiring programmer. By the end of this article, you’ll have a solid understanding of variables, boolean variables and operators, while loops, for loops, if statements, and code indentation in Python. The Jupyter notebook file associated with this blog post, which includes all of this information, can be found here. We encourage you to code along in your own Jupyter notebook file and use the linked notebook as a guideline.
Prerequisites
Variables
Variables are the basic units of storage in any programming language. In Python, you can create a variable simply by assigning a value to a name:
x = 10
name = "Alice"
Variables can store different types of data, including numbers, strings, lists, and more.
Data Types in Python
Integers: Whole numbers without a decimal point.
age = 25
Floats: Numbers with a decimal point.
temperature = 98.6
Strings: Sequences of characters enclosed in quotes.
greeting = "Hello, World!"
Lists: Ordered collections of items, which can be of different types.
fruits = ["apple", "banana", "cherry"]
Tuples: Ordered collections of items, which are immutable (cannot be changed).
coordinates = (10.0, 20.0)
Dictionaries: Collections of key-value pairs.
person = {"name": "Alice", "age": 30}
Sets: Unordered collections of unique items.
unique_numbers = {1, 2, 3, 4, 5}
Booleans: True or False values.
is_student = True
Boolean Variables and Operators
Boolean variables store True or False values. These are especially useful in conditional statements and loops. Boolean operators allow you to perform logical operations:
is_raining = True
is_sunny = False
# Boolean operators
print(is_raining and is_sunny) # False
print(is_raining or is_sunny) # True
print(not is_raining) # False
While Loop
A while loop allows you to repeat a block of code as long as a condition is true. This is useful for tasks that require repeated actions until a certain condition is met.
count = 0
while count < 5:
print("Counting:", count)
count += 1
In this example, the loop prints the count variable and increments it until the condition count < 5
is no longer true.
For Loop
For loops are used for iterating over a sequence (such as a list, tuple, or string) and executing a block of code for each element.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
This loop prints each fruit in the list.
If Statement
If statements allow you to execute a block of code based on whether a condition is true or false.
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is 5 or less")
In this example, the if statement checks if ‘x
‘ is greater than 5 and prints a message accordingly.
Code Indentation in Python
Python uses indentation to define the scope of loops, functions, and conditional statements. Proper indentation is crucial for your code to run correctly.
if x > 5:
print("This is indented")
if x > 10:
print("This is further indented")
Each level of indentation represents a new block of code. Consistency in using either spaces or tabs for indentation is important to avoid errors.
Conclusion
Understanding these foundational programming concepts is crucial as you continue your Python journey. Mastering variables, boolean operators, loops, if statements, and proper indentation will provide a strong base for more advanced topics. Keep practicing, and you’ll become proficient in no time!