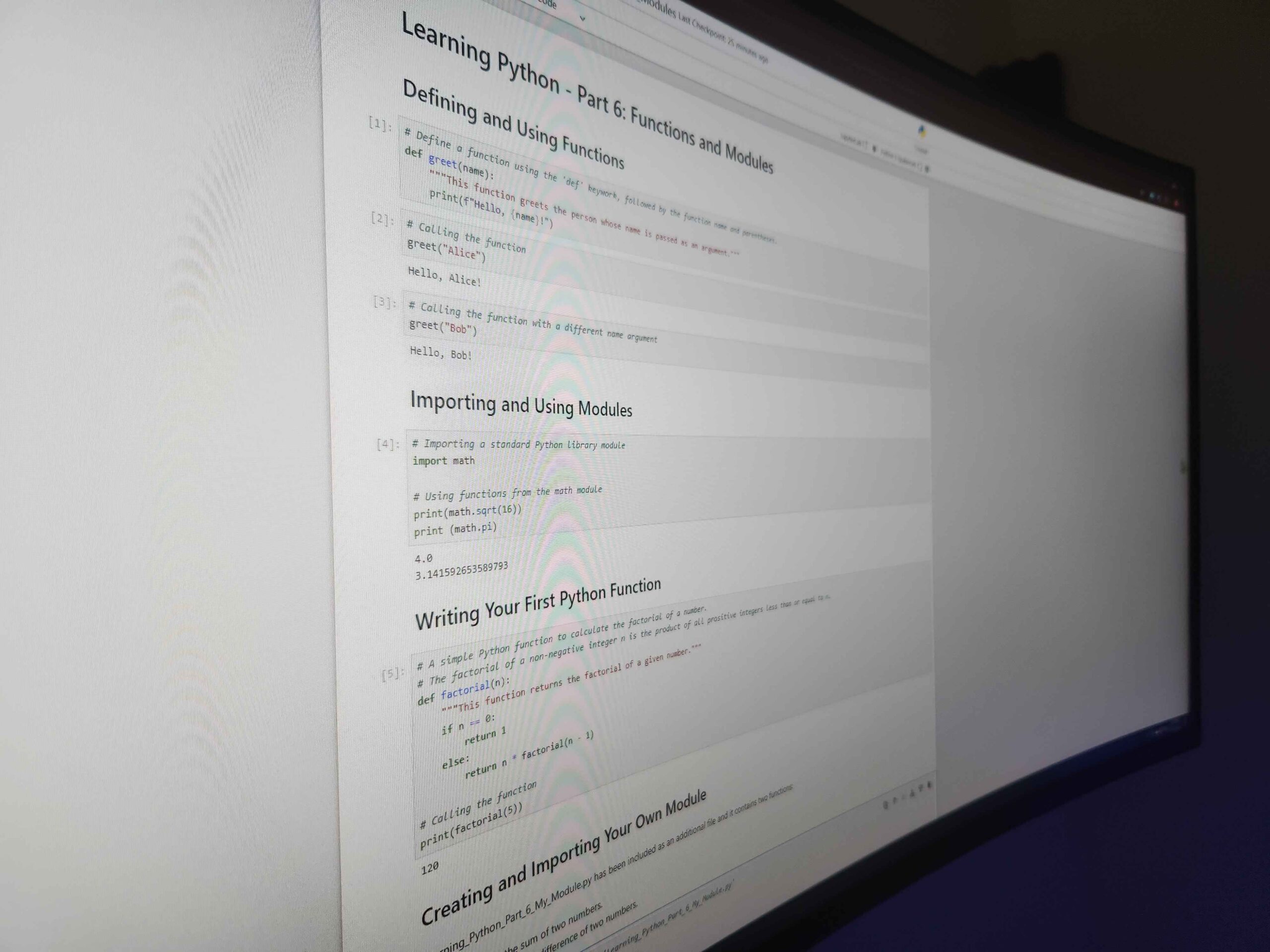
Welcome to Part 6 of our “Learning Python” series! In this post, we will explore two fundamental concepts in Python programming: functions and modules. Functions allow you to encapsulate reusable pieces of code, making your programs more modular and easier to manage. Modules enable you to organize your code and reuse it across different projects. By mastering these concepts, you’ll be well-equipped to write clean, efficient, and maintainable Python code. The Jupyter notebook file associated with this blog post, which includes all of this information, can be found here. We encourage you to code along in your own Jupyter notebook file and use the linked notebook as a guideline.
Defining and Using Functions
Functions are blocks of code that perform a specific task. They help you avoid code duplication and make your programs more readable. You define a function using the def
keyword, followed by the function name and parentheses.
def greet(name):
"""This function greets the person whose name is passed as an argument."""
print(f"Hello, {name}!")
# Calling the function
greet("Alice") # Output: Hello, Alice!
In this example, we define a function greet
that takes a parameter name
and prints a greeting message. The function is then called with the argument "Alice"
.
Importing and Using Modules
Modules are files containing Python code that can be imported and used in other Python programs. Python has a rich standard library of modules, and you can also create your own.
# Importing a standard library module
import math
# Using functions from the math module
print(math.sqrt(16)) # Output: 4.0
print(math.pi) # Output: 3.141592653589793
In this example, we import the math
module from the Python standard library and use its sqrt
function to calculate the square root of 16 and the pi
constant.
Writing Your First Python Function
Let’s write a simple Python function to calculate the factorial of a number. The factorial of a non-negative integer n is the product of all positive integers less than or equal to n.
def factorial(n):
"""This function returns the factorial of a given number."""
if n == 0:
return 1
else:
return n * factorial(n - 1)
# Calling the function
print(factorial(5)) # Output: 120
In this example, we define a recursive function factorial
that calculates the factorial of a given number n
. The function calls itself with the argument n-1
until it reaches the base case n == 0
.
Creating and Importing Your Own Module
To demonstrate how to create and import your own module, let’s create a module named mymodule.py
containing two simple functions.
mymodule.py:
def add(a, b):
"""This function returns the sum of two numbers."""
return a + b
def subtract(a, b):
"""This function returns the difference of two numbers."""
return a - b
You can now import and use the functions from mymodule.py
in another Python script.
# Importing our custom module
import mymodule
# Using functions from the custom module
print(mymodule.add(10, 5)) # Output: 15
print(mymodule.subtract(10, 5)) # Output: 5
In this example, we import our custom module mymodule
and use its add
and subtract
functions to perform simple arithmetic operations.
Conclusion
In this part of our “Learning Python” series, we covered the basics of Python functions and modules. We learned how to define and use functions, import and use modules from the Python standard library, and create our own modules. These concepts are crucial for writing modular and maintainable Python code. By mastering functions and modules, you’ll be able to organize your code more effectively and reuse it across different projects.
Thank you for following along and farewell for now!